-
Notifications
You must be signed in to change notification settings - Fork 479
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Add support for Tornado 16X SQ air conditioner (0x4E2A) #520
Merged
Merged
Changes from 9 commits
Commits
Show all changes
15 commits
Select commit
Hold shift + click to select a range
f02c9ab
Add support for Tornado 16X SQ air conditioner
felipediel f4707c0
Make Tornado a generic HVAC class
felipediel 32d7186
Better names
felipediel 1d357e2
Clean up IntEnums
felipediel 179133c
Clean up encoders
felipediel 9a366e5
Fix indexes
felipediel 34c95a6
Improve set_state() interface
felipediel 0e44f71
Enumerate presets
felipediel 1ef9eec
Rename state to power in get_ac_info()
felipediel 855254e
Merge remote-tracking branch 'upstream/dev' into hvac-support
felipediel bcde9f6
Paint it black
felipediel d97f887
Use CRC16 helper class
felipediel f840d5d
Remove log messages
felipediel fbc0f01
Fix bugs
felipediel b07de4c
Return state in set_state()
felipediel File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I might be misunderstanding what you mean.
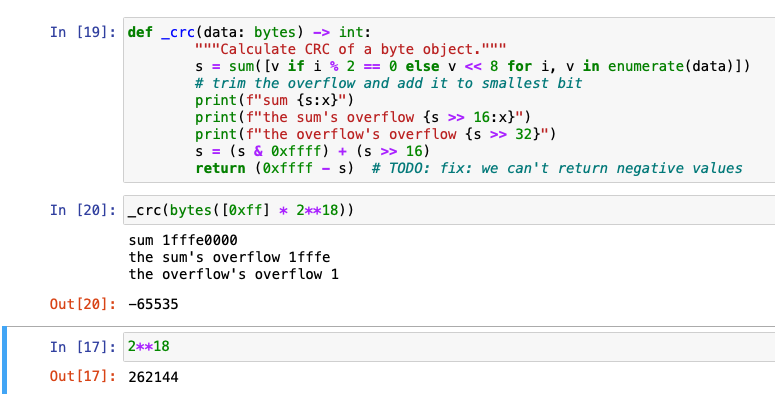
But if you mean be negative like in the screenshot, I think it'd be okay just to check that the packet is smaller than 2^18B.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Yes, overflow is unlikely as we are not dealing with packets of this size.
& 0xFFFF
should be enough as a safeguard:There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I just found the polynomial, now we can do: