-
Notifications
You must be signed in to change notification settings - Fork 7
/
convert.py
159 lines (132 loc) · 5.17 KB
/
convert.py
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
#!/usr/bin/bash
import os
import re
import shutil
from datetime import datetime
from fnmatch import fnmatch
import aliyun_oss
ROOT = '.'
SOURCE_FOLDERS = ['java', 'rabbitmq', 'rocketmq', 'other']
# SOURCE_FOLDERS = ['distributed']
OUTPUT_FOLDER = '_output'
DOCS_FOLDER = os.path.join('docs', 'src')
FILE_PATTERN = '\d{8}.*\.md'
PREFIX = '''---
title: {title}
author: Scarb
date: {date}
---
原文地址:[{address}]({address})
'''
SUFFIX = '''
---
欢迎关注公众号【消息中间件】(middleware-mq),更新消息中间件的源码解析和最新动态!
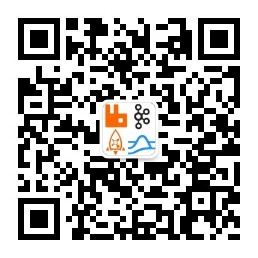
'''
def find_first_line_start_with_in_file(file_path):
"""
find first line start with '#' in file
:param f:
:return:
"""
with open(file_path, 'r', encoding='UTF-8') as f:
lines = f.readlines()
for line in lines:
if line.startswith('# '):
return line[2:]
def _replace_image_path_to_github(line):
pattern = r'!\[(.*)\]\(\.\./(assets/.+)\)'
re_img_url = re.compile(pattern)
match = re_img_url.match(line)
if match:
l = re.sub(pattern=pattern,
repl='', string=line)
return l
pattern = r'\[(\d+)\]: \.\./(assets/.+)'
re_img_url = re.compile(pattern)
match = re_img_url.match(line)
if match:
l = re.sub(pattern=pattern,
repl='[\\1]: https://raw.githubusercontent.com/HScarb/knowledge/master/\\2', string=line)
return l
return line
def _upload_local_image_to_oss(line):
if line.startswith('[TOC]'):
return '[[toc]]' + os.linesep
# upload image in format like: ``
pattern = r'!\[(.*)\]\(\.\./(assets/.+)\)'
re_img_url = re.compile(pattern)
match = re_img_url.match(line)
if match:
repl = ''.format(aliyun_oss.upload_to_oss(match.groups()[1]))
l = re.sub(pattern=pattern, repl=repl, string=line)
return l
# upload image in reference pattern like: ![1] ...
pattern = r'\[(\d+)\]: \.\./(assets/.+)'
re_img_url = re.compile(pattern)
match = re_img_url.match(line)
if (match):
repl = '[\\1]: {}'.format(aliyun_oss.upload_to_oss(match.groups()[1]))
l = re.sub(pattern=pattern, repl=repl, string=line)
return l
return line
def convert_file_with_lambda(file_path, output_path, fn, prefix='', suffix=''):
"""
Replace local image url in markdown to github repo url
:param file_path: file path of markdown file
"""
with open(file_path, 'r', encoding='UTF-8') as f, open(output_path, 'w', encoding='UTF-8') as f_out:
lines = f.readlines()
f_out.write(prefix)
for line in lines:
f_out.write(fn(line))
f_out.write(suffix)
def recreate_dir(dir):
try:
shutil.rmtree(dir)
except:
pass
os.mkdir(dir)
def generate_readme_for_dir(dir, folder):
"""
generate vuepress README.md file for dir
:return:
"""
readme_path = os.path.join(dir, 'README.md')
print(readme_path)
with open(readme_path, 'w', encoding='UTF-8') as f:
f.writelines('# {title}\n\n'.format(title=folder))
for path, subdirs, files in os.walk(folder):
for name in files:
if not name.startswith('2'):
continue
title = find_first_line_start_with_in_file(os.path.join(DOCS_FOLDER, folder, name)).strip()
f.writelines('[{title}]({path})\n\n'.format(title=title, path=name))
def convert_image_url_to_output():
"""
convert local image path to github url
and export to _output folder
:return:
"""
for folder in SOURCE_FOLDERS:
recreate_dir(os.path.join(OUTPUT_FOLDER, folder))
recreate_dir(os.path.join(DOCS_FOLDER, folder))
for path, subdirs, files in os.walk(folder):
for name in files:
if re.match(r'\d{8}.+?\.md', name):
# if fnmatch(name, FILE_PATTERN):
file_path = os.path.join(path, name)
output_path = os.path.join(OUTPUT_FOLDER, path, name)
docs_path = os.path.join(DOCS_FOLDER, path, name)
print('------' + file_path + '------')
convert_file_with_lambda(file_path, output_path, _replace_image_path_to_github, suffix=SUFFIX)
convert_file_with_lambda(file_path, docs_path, _upload_local_image_to_oss,
prefix=PREFIX.format(
title=find_first_line_start_with_in_file(file_path).strip(),
date=datetime.strptime(name[0:8], '%Y%m%d').strftime('%Y-%m-%d'),
address='http://hscarb.github.io/' + file_path.replace('\\', '/').replace('.md', '.html')
),
suffix=SUFFIX)
generate_readme_for_dir(os.path.join(DOCS_FOLDER, folder), folder)
if __name__ == '__main__':
convert_image_url_to_output()